boardInput.jsx
import React, { useEffect, useRef, useState } from 'react';
import axios from 'axios';
import '../css/board.css'
const BoardInput = () => {
const seqRef = useRef(1);
const [boardDTO, setBoardDTO] = useState({
//초기값 설정
name: '',
sunject:'',
content:''
});
const { name, subject, content } = boardDTO;
const [nameDiv, setNameDiv] = useState('');
const [subjectDiv, setSubjectDiv] = useState('');
const [contentDiv, setContentDiv] = useState('');
const onInput = (e) => {
const { name, value } = e.target;
setBoardDTO({
...boardDTO,
[name] : value
});
}
const onWriteSubmit = (e) => {
e.preventDefault();
setNameDiv('');
setSubjectDiv('');
setContentDiv('');
if(!name){
setNameDiv('작가 입력');
return;
}
if(!subject){
setSubjectDiv('제목 입력');
return;
}
if(!content){
setContentDiv('내용 입력');
return;
}
setBoardDTO({
...boardDTO,
//seq: seqRef.current++
});
axios.post('http://localhost:9000/board2/boardInput', boardDTO)
.then(res => {
alert('글쓰기 완료')
});
}
return (
<div>
<form className='BoardInputForm' onSubmit={ onWriteSubmit }>
<table border='1' cellPadding='7' cellSpacing='0'>
<thead></thead>
<tbody>
<tr>
<th width='100'>작가</th>
<td>
<input
type='text'
name='name'
value={ name }
onChange={ onInput }
/>
<div className='nameDiv'>{ nameDiv }</div>
</td>
</tr>
<tr>
<th>제목</th>
<td>
<input
type='text'
name = 'subject'
value={ subject }
size = '50'
onChange={ onInput }
/>
<div className='subjectDiv'>{ subjectDiv }</div>
</td>
</tr>
<tr>
<th>내용</th>
<td>
<textarea
name='content'
value={ content }
cols='50'
rows='15'
onChange={ onInput }
/>
<div className='contentDiv'>{ contentDiv }</div>
</td>
</tr>
<tr>
<td colSpan='2' align='center'>
<button>게시글 추가</button>
<button type='reset'>새로 작성</button>
</td>
</tr>
</tbody>
</table>
</form>
</div>
);
};
export default BoardInput;
seq 는 자꾸 값 이상하게 나와서 강사님이 패스함
boardList.jsx는 따로 수업에서 안하고 넘어감
Chapter01HelloGradle 에서 board2Controller
package board.controller;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import board.bean.BoardDTO;
@CrossOrigin(origins = "http://localhost:3000")
@RestController
public class Board2Controller {
private List<BoardDTO> list = new ArrayList<>();
@PostMapping(value = "/board2/boardInput")
public void boardInput(
@RequestBody BoardDTO boardDTO) {
//boardDTO.setLogtime(new SimpleDateFormat("yyyy.MM.dd").format(new Date()));
boardDTO.setLogtime(new Date());
System.out.println(boardDTO);
list.add(boardDTO);
}
}
주석친건 logtime은 Date 타입인데 new SimpleDateFormat한건 String이라 타입 일치해줘야하는데 일단 주석
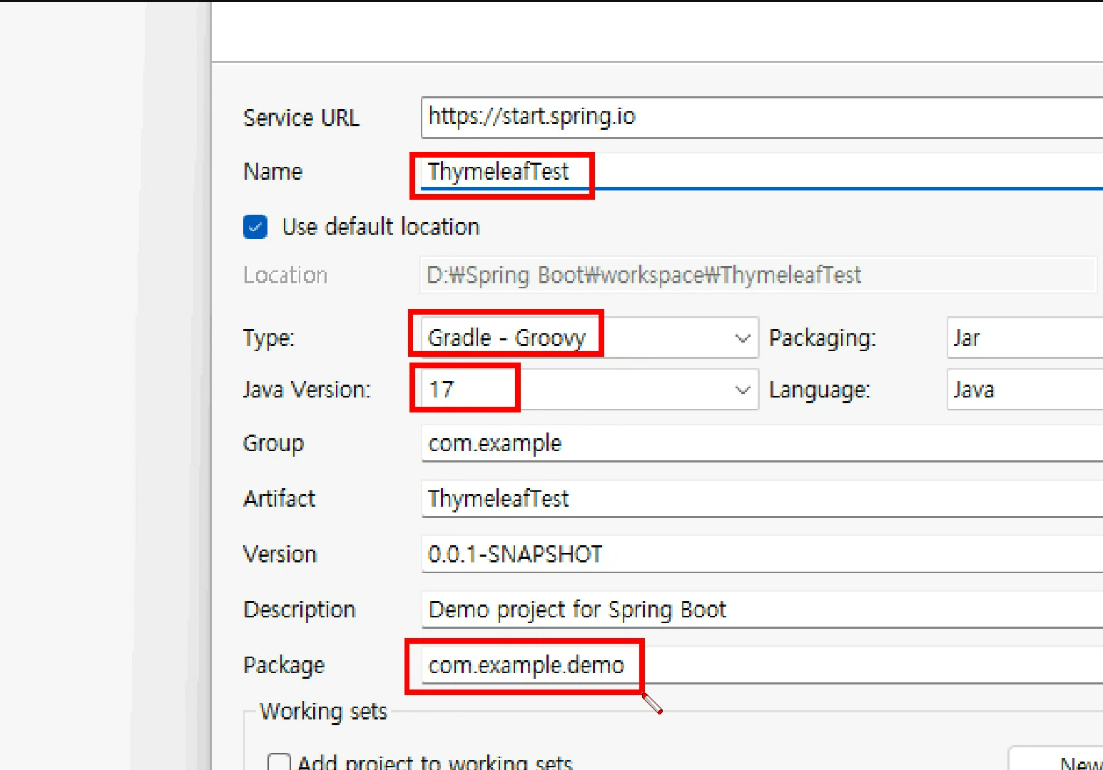


이클립스 마켓 ㄱㄱ



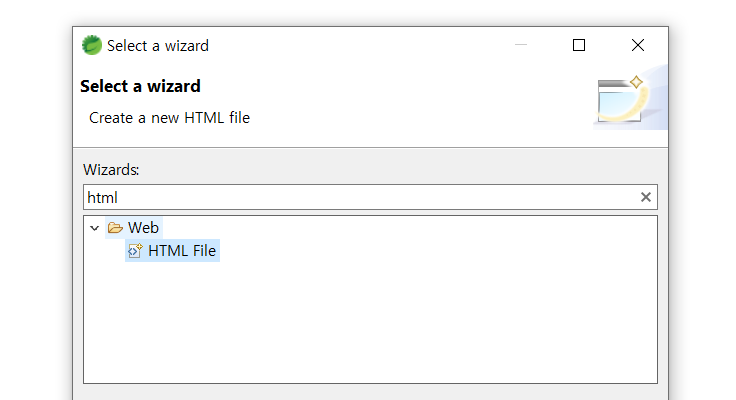
이제 새로운 파일 new로 추가할 때 html 형식도 뜸
나머지는 ThymeleafTest 프로젝트에서 진행
src/main/java
src/main/resources
두개만 건드림 자세한건 압축파일 ThymeleafTest 참조
resources에서 static에는 자바스크립트 , css , image 등 정적 파일
template에는 html 파일이 들어간다.
타임리프 문법만 익히면 됨 jsp랑 비슷함
둘다 서버사이드 랜더링